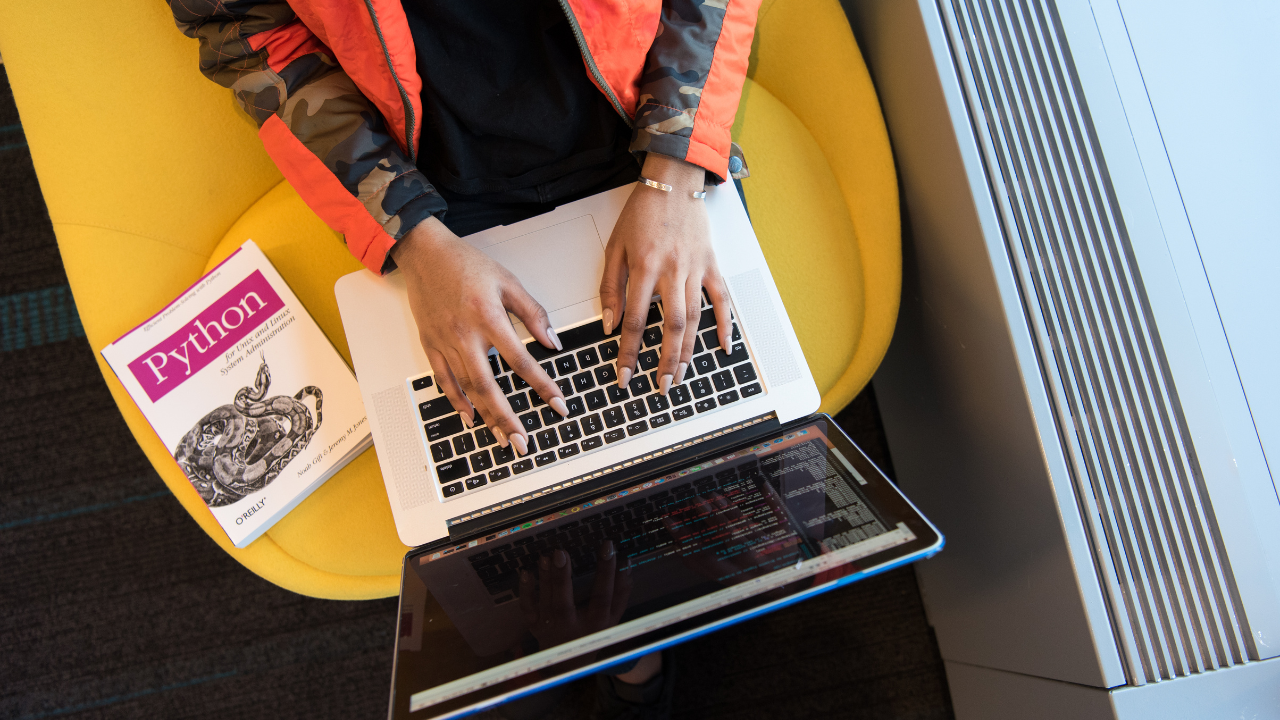
Master Python Metaclasses for Advanced Class Customization
By Alyce Osbourne
Master Python Metaclasses for Advanced Class Customization
Have you ever wondered why you can define some names and numbers in an Enum, and it magically becomes a different object with the methods you defined? Or how do abstract classes force you to implement their methods?
The answer is: metaclasses!
What is a metaclass?
To understand metaclasses, we need to understand that in Python, everything is an object, including classes. Just as classes are blueprints for creating objects, metaclasses are blueprints for creating classes. In simpler terms, a metaclass defines how classes behave.
The purpose of metaclasses
Metaclasses serve several purposes, the most common being:
- Class Customization: Metaclasses allow you to modify or extend class definitions during creation.
- Enforcing Coding Standards: They can enforce certain coding standards or patterns within your classes.
- Automatic Registration: Useful for creating registries where all subclasses of a given class are automatically registered.
- Dependency Injection: Facilitating dependency injection mechanisms at the class level.
Basic syntax and usage
Here’s a simple example of a metaclass in Python:
class Meta(type):
def __new__(cls, name: str, bases:tuple[type], dct: dict):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=Meta):
pass
When MyClass
is defined, the metaclass Meta
prints a message, demonstrating that it intervenes during class creation.
Use cases
1. Class customization
Metaclasses can modify class attributes or methods dynamically:
class AttributeModifier(type):
def __new__(cls, name: str, bases: tuple[type], dct: dict):
dct['new_attribute'] = 'Hello, Metaclasses!'
return super().__new__(cls, name, bases, dct)
class CustomClass(metaclass=AttributeModifier):
pass
print(CustomClass.new_attribute) # Output: Hello, Metaclasses!
2. Enforcing coding standards
Metaclasses can ensure that classes adhere to specific rules, such as having certain methods or attributes:
class InterfaceEnforcer(type):
def __new__(cls, name: str, bases: tuple[type], dct: dict):
if 'required_method' not in dct:
raise TypeError("Classes must define 'required_method'")
return super().__new__(cls, name, bases, dct)
class ConformingClass(metaclass=InterfaceEnforcer):
def required_method(self):
pass
class NonConformingClass(metaclass=InterfaceEnforcer):
pass # This will raise a TypeError
3. Automatic registration
Metaclasses can be used to automatically register all subclasses of a base class:
registry = {}
class AutoRegister(type):
def __new__(cls, name: str, bases: tuple[type], dct: dict):
new_class = super().__new__(cls, name, bases, dct)
registry[name] = new_class
return new_class
class BaseClass(metaclass=AutoRegister):
pass
class SubClass(BaseClass):
pass
print(registry) # Output: {'BaseClass': <class '__main__.BaseClass'>, 'SubClass': <class '__main__.SubClass'>}
Built-in metaclasses in Python
Several built-in classes and features in Python use their own metaclasses to define and customize their behavior. Here are some notable examples:
1. type
The type
itself is a metaclass. It’s the default metaclass in Python and is used to create all classes and types:
class MyClass:
pass
print(type(MyClass)) # <class 'type'>
If we wish to create a metatype, we do so by subclassing the type
metaclass.
2. ABCMeta
The ABCMeta
is the metaclass for defining Abstract Base Classes (ABCs). It provides mechanisms for defining abstract methods and ensuring they are implemented by subclasses:
from abc import ABC, abstractmethod
class MyABC(ABC):
@abstractmethod
def my_method(self):
pass
class MyClass(MyABC):
def my_method(self):
return "Implemented!"
class IncompleteClass(MyABC): # raises an error
pass
ABC
s make use of metaclasses to enforce the implementation of abstract methods at the time the class is created, much like the above example where we enforce standards.
3. EnumMeta
The EnumMeta
is used to create enumerations in Python. It is the metaclass for the Enum
class:
from enum import Enum
class Color(Enum):
RED = 1
GREEN = 2
BLUE = 3
print(Color.RED) # Output: Color.RED
This is by far the most interesting and magical-seeming use of metaclasses in the standard library.
It enables the class variables to be converted into instances of a type, usually Enum, seemingly by magic. These objects then have all of the methods we have implemented in the enums body.
How this works is that the class variables are treated as the name and arguments to instantiate an instance of Enum (or the class you mix with it using multiple inheritance).
When should I use a metaclass?
The short answer? Almost never. Metaclasses fundamentally alter how classes are created and behave, and outside of defining specialized types, this is rarely a feature the average developer will need to reach for. There are some instances where a specialized type might be desired, such as when writing code to interface with external data services, such as in the implementation of ORMs, but these are generally rare since most functionality can be defined as a normal class. But in those rare cases you do find you need them, such as writing adapters into other software, they can save you many hours of work!
Final thoughts
Using metaclasses is something to approach thoughtfully, with care and purpose in mind. They have the potential to significantly change how a class operates by influencing how classes are created. Because this level of control is typically not needed in our everyday programming tasks, the use of metaclasses is not very common. Understanding them can help you identify the rare occasions where their use is desirable.