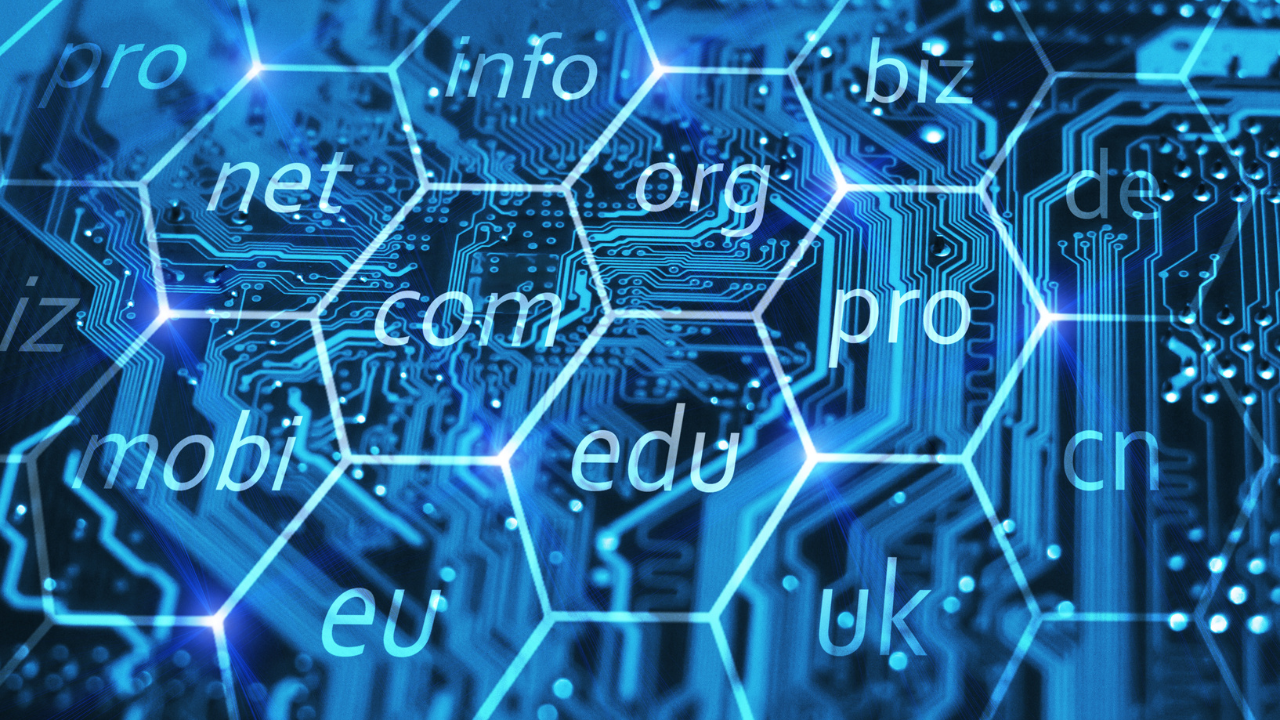
Web Scraping and URL Parsing Made Easy with Python’s urllib
By Alyce Osbourne
Python’s urllib
module is a powerful and versatile library for working with URLs and handling various internet-related tasks. It provides a range of functionalities, from fetching data from the web to parsing URLs. In this blog post, we’ll explore the key features of the urllib
module, covering its submodules and demonstrating practical examples to help you master its usage.
Introduction to urllib
The urllib
module is a collection of modules for working with URLs, consisting of several submodules:
urllib.request
: For opening and reading URLs.urllib.parse
: For parsing URLs.urllib.error
: Contains the exceptions raised byurllib.request
.urllib.robotparser
: For parsing robots.txt files.
We’ll focus on the most commonly used submodules: urllib.request
and urllib.parse
.
Fetching data from the Web
Using urllib.request
To fetch data from the web, you can use the urllib.request
module. Here’s a basic example of how to use it:
import urllib.request
url = "<http://example.com>"
response = urllib.request.urlopen(url)
data = response.read()
print(data.decode('utf-8'))
This code snippet opens the URL, reads the content, and prints it. urlopen
returns an HTTPResponse object, which you can use to read the data.
Handling HTTP errors
When working with web requests, you may encounter HTTP errors. The urllib.error
module provides specific exceptions to handle these errors:
from urllib.error import URLError, HTTPError
try:
response = urllib.request.urlopen(url)
data = response.read()
except HTTPError as e:
print(f'HTTP error: {e.code} {e.reason}')
except URLError as e:
print(f'URL error: {e.reason}')
This code catches and handles both HTTP-specific errors and general URL errors.
Working with headers
You can also send custom headers with your requests:
req = urllib.request.Request(url, headers={'User-Agent': 'Mozilla/5.0'})
response = urllib.request.urlopen(req)
data = response.read()
print(data.decode('utf-8'))
Adding headers can be useful for mimicking browser behaviour or accessing APIs that require specific headers.
Parsing URLs with urllib.parse
The urllib.parse
module is essential for parsing and manipulating URLs. It provides functions to split URLs into components, join components into URLs, and more.
Parsing a URL
from urllib.parse import urlparse
url = "<http://example.com/path?query=param#fragment>"
parsed_url = urlparse(url)
print(parsed_url.scheme) # 'http'
print(parsed_url.netloc) # 'example.com'
print(parsed_url.path) # '/path'
print(parsed_url.query) # 'query=param'
print(parsed_url.fragment) # 'fragment'
Constructing a URL
You can construct a URL from components using urlunparse
:
from urllib.parse import urlunparse
components = ('http', 'example.com', '/path', '', 'query=param', 'fragment')
url = urlunparse(components)
print(url) # '<http://example.com/path?query=param#fragment>'
Handling URL encodings
URL encoding is crucial when dealing with special characters in URLs. The urllib.parse
module provides functions for encoding and decoding URLs.
Encoding a URL
from urllib.parse import quote
query = "Hello World!"
encoded_query = quote(query)
print(encoded_query) # 'Hello%20World%21'
Decoding a URL
from urllib.parse import unquote
decoded_query = unquote(encoded_query)
print(decoded_query) # 'Hello World!'
Managing Cookies with http.cookiejar
and urllib.request
Cookies are essential for maintaining sessions on the web. You can manage cookies using http.cookiejar
and urllib.request
.
Handling Cookies
import http.cookiejar
import urllib.request
cookie_jar = http.cookiejar.CookieJar()
opener = urllib.request.build_opener(urllib.request.HTTPCookieProcessor(cookie_jar))
response = opener.open(url)
for cookie in cookie_jar:
print(f'Cookie: {cookie.name}={cookie.value}')
This code snippet demonstrates how to create a cookie jar, attach it to an opener, and handle cookies during requests.
Final thoughts
The urllib
module is a powerful tool for working with URLs in Python. Whether you’re fetching data from the web, parsing URLs, handling encodings, or managing cookies, urllib
has you covered. With the knowledge and examples provided in this guide, you should be well-equipped to handle a wide range of internet-related tasks in your Python projects.